Custom Templates
Although there are built-in templates, it will never be enough to cover all the scenanrios.
Pinferencia supports custom templates. It's easy to customize a template and use it in your service.
First let's try to create a simple template:
- Input a list of numbers.
- Display the mean, max and min of the numbers.
Model
The model is simple, and the service can be defined as:
app.py |
---|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15 | from typing import List
from pinferencia import Server
def stat(data: List[float]) -> dict:
return {
"mean": sum(data) / len(data),
"max": max(data),
"min": min(data),
}
service = Server()
service.register(model_name="stat", model=stat, metadata={"task": "Stat"})
|
Template
Pinferencia provides a BaseTemplate
to extend on to build a custom template.
First, we create a JSON input field and display field in two columns.
frontend.py |
---|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27 | import streamlit as st
from pinferencia.frontend.app import Server
from pinferencia.frontend.templates.base import BaseTemplate
class StatTemplate(BaseTemplate):
title = (
'<span style="color:salmon;">Numbers</span> '
'<span style="color:slategray;">Statistics</span>'
)
def render(self):
super().render()
json_template = "[]"
col1, col2 = st.columns(2)
col2.write("Request Preview")
raw_text = col1.text_area("Raw Data", value=json_template, height=150)
col2.json(raw_text)
backend_address = "http://127.0.0.1:8000"
service = Server(
backend_server=f"{backend_address}",
custom_templates={"Stat": StatTemplate},
)
|
Start the Service
$ pinfer sum_mnist:service --frontend-script=sum_mnist_frontend.py
Pinferencia: Frontend component streamlit is starting...
Pinferencia: Backend component uvicorn is starting...
And open the browser you will see:
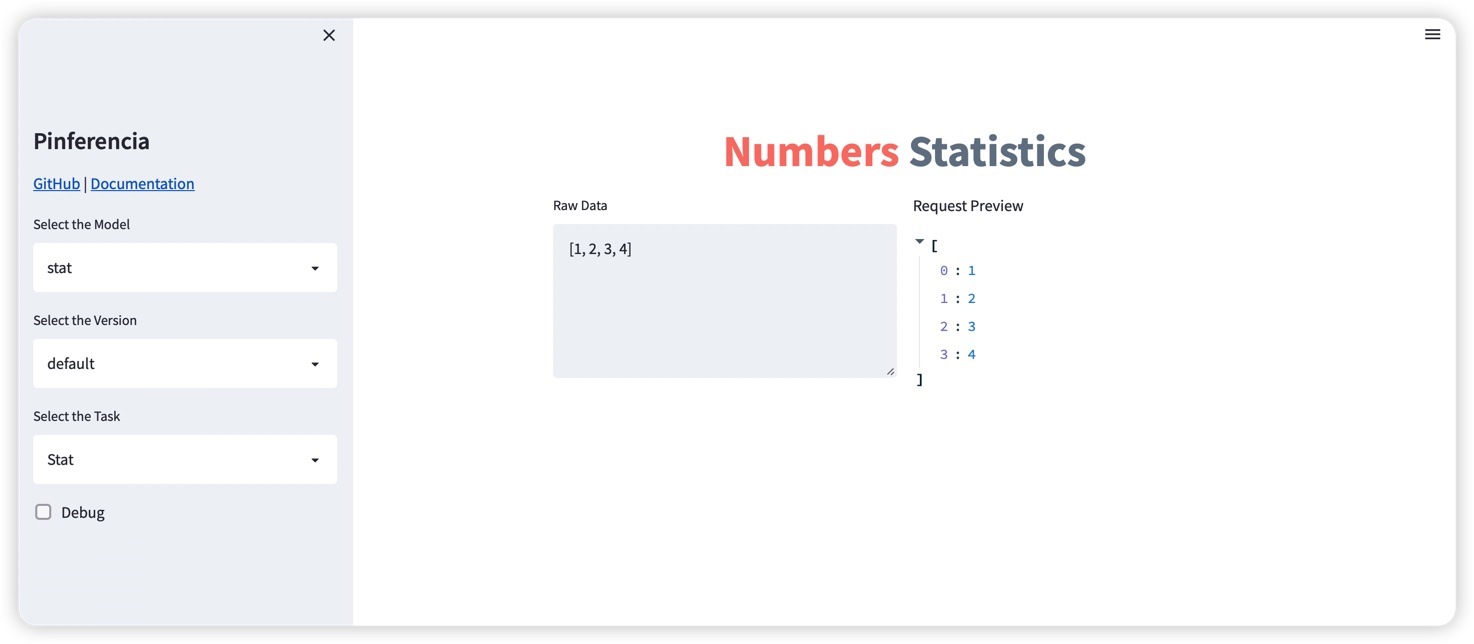
Call Backend and Display Results
Add the below highlighted codes to send request to backend and display the result.
frontend.py |
---|
1
2
3
4
5
6
7
8
9
10
11
12
13
14
15
16
17
18
19
20
21
22
23
24
25
26
27
28
29
30
31
32
33
34
35
36
37
38
39
40 | import json
import streamlit as st
from pinferencia.frontend.app import Server
from pinferencia.frontend.templates.base import BaseTemplate
class StatTemplate(BaseTemplate):
title = (
'<span style="color:salmon;">Numbers</span> '
'<span style="color:slategray;">Statistics</span>'
)
def render(self):
super().render()
json_template = "[]"
col1, col2 = st.columns(2)
col2.write("Request Preview")
raw_text = col1.text_area("Raw Data", value=json_template, height=150)
col2.json(raw_text)
pred_btn = st.button("Run") # (1)
if pred_btn:
with st.spinner("Wait for result"): # (2)
prediction = self.predict(json.loads(raw_text)) # (3)
st.write("Statistics")
result_col1, result_col2, result_col3 = st.columns(3) # (4)
result_col1.metric(label="Max", value=prediction.get("max"))
result_col2.metric(label="Min", value=prediction.get("min"))
result_col3.metric(label="Mean", value=prediction.get("mean"))
backend_address = "http://127.0.0.1:8000"
service = Server(
backend_server=f"{backend_address}",
custom_templates={"Stat": StatTemplate},
)
|
- Display a button to trigger prediction.
- Display a spinner while sending the request.
- Send the data to backend.
- Display the results in 3 columns.
Start the Service again, and You Will Get:
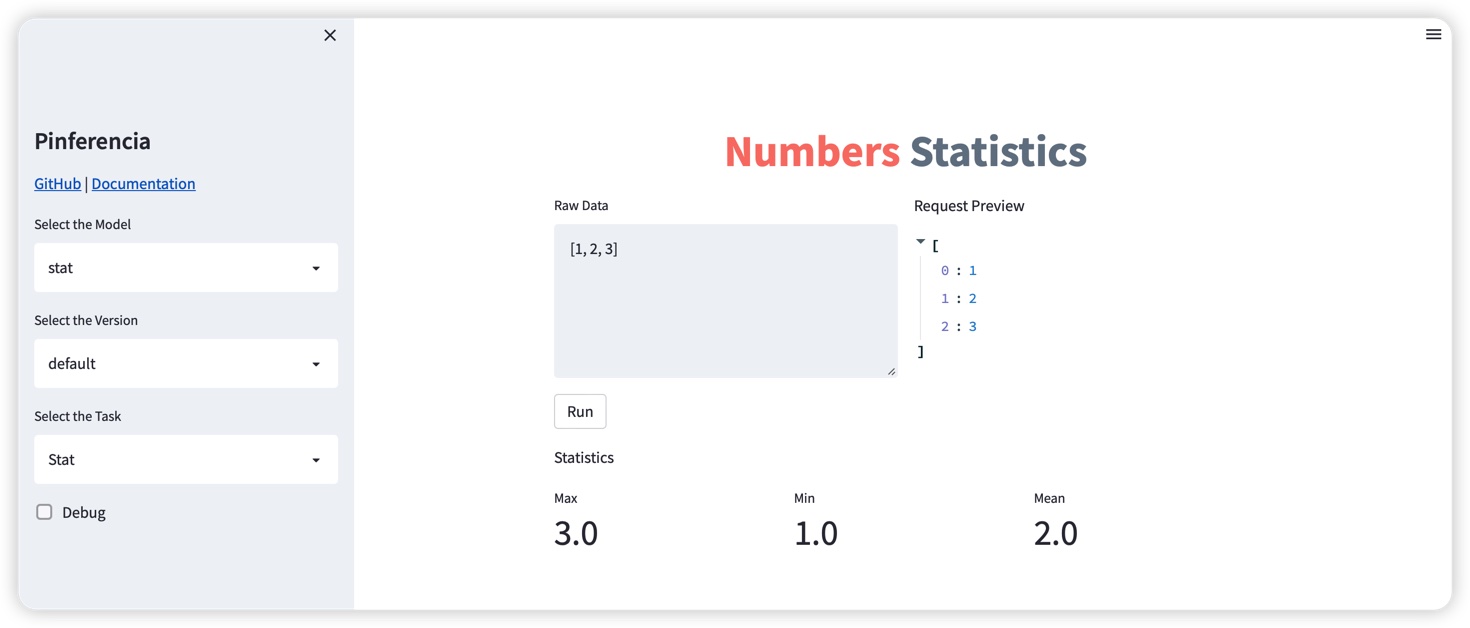